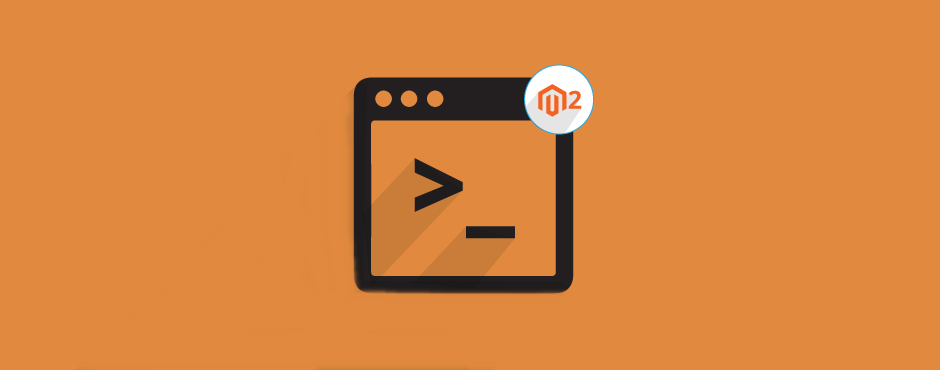
The custom console commands in Magento 2 are very useful when you need to perform tasks that are repetitive and are to be done in the same manner every time!
Here, we have taken a simple example to display a message when a custom Magento 2 CLI command is run:
Step 1: Define command in di.xml
In di.xml
file, you can use a type with name Magento\Framework\Console\CommandList
to define the command option.
File: app/code/Mageclues/HelloWorld/etc/di.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magento\Framework\Console\CommandList">
<arguments>
<argument name="commands" xsi:type="array">
<item name="examplePingHello" xsi:type="object">Mageclues\HelloWorld\Console\Pinghello</item>
</argument>
</arguments>
</type>
</config>
This config will declare a command class Pinghello
. This class will define the command name and execute()
method for this command.
Step 2: Create command class
As define in di.xml, we will create a command class:
File: app/code/Mageclues/HelloWorld/Console/Pinghello.php
<?php
namespace Mageclues\HelloWorld\Console;
use Symfony\Component\Console\Command\Command;
use Symfony\Component\Console\Input\InputInterface;
use Symfony\Component\Console\Output\OutputInterface;
class Pinghello extends Command
{
protected function configure()
{
$this->setName('example:pinghello');
$this->setDescription('Example command line');
parent::configure();
}
protected function execute(InputInterface $input, OutputInterface $output)
{
$output->writeln("Hello MageClues!");
}
}
n this function, we will define 2 methods:
configure()
method is used to set the name, description, command line arguments of the magento 2 add command line.execute()
method will run when we call this command line via console.
After declare this class, please flush Magento cache and type this command:
php bin/magento --list
Now you can run php bin/magento example:pinghello
from the command to see the result.
Now, we will look how to add the arguments for the command.
<?php
namespace Mageclues\HelloWorld\Console;
use Symfony\Component\Console\Command\Command;
use Symfony\Component\Console\Input\InputInterface;
use Symfony\Component\Console\Output\OutputInterface;
use Symfony\Component\Console\Input\InputOption;
class Pinghello extends Command
{
const NAME = 'name';
protected function configure()
{
$options = [
new InputOption(self::NAME, null, InputOption::VALUE_REQUIRED, 'Name')
];
$this->setName('example:pinghello')
->setDescription('Example command line')
->setDefinition($options);
parent::configure();
}
protected function execute(InputInterface $input, OutputInterface $output)
{
if ($name = $input->getOption(self::NAME)) {
$output->writeln("Hello " . $name);
} else {
$output->writeln("Hello MageClues!");
}
return $this;
}
}
We defined name argument for command line in configure() function and get it in execute() function. Please clear cache and run php bin/magento example:pinghello –name=”Magento2″ from the command line to check the result. It will show “Hello Magento2”