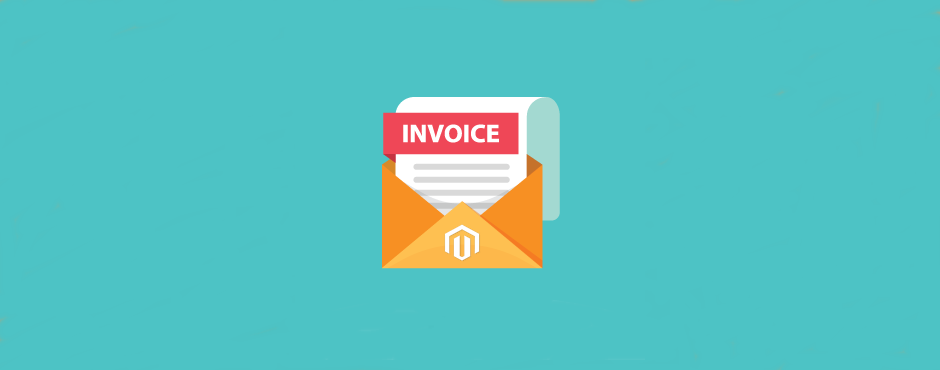
In article, we will learn how to create Invoice programmatically.
To Create Invoice of an order-
<?php
namespace Mageclues\Invoice\Controller;
class CreateInvoice extends Magento\Framework\App\Action\Action
{
/**
* @var \Magento\Sales\Api\OrderRepositoryInterface
*/
protected $_orderRepository;
/**
* @var \Magento\Sales\Model\Service\InvoiceService
*/
protected $_invoiceService;
/**
* @var \Magento\Framework\DB\Transaction
*/
protected $_transaction;
public function __construct(
\Magento\Framework\App\Action\Context $context,
\Magento\Sales\Api\OrderRepositoryInterface $orderRepository,
\Magento\Sales\Model\Service\InvoiceService $invoiceService,
\Magento\Framework\DB\Transaction $transaction
) {
$this->_orderRepository = $orderRepository;
$this->_invoiceService = $invoiceService;
$this->_transaction = $transaction;
parent::__construct($context);
}
/**
* Marketplace order invoice controller.
*
* @return \Magento\Framework\View\Result\Page
*/
public function execute()
{
$orderId = 100;
$order = $this->_orderRepository->get($orderId);
if($order->canInvoice()) {
$invoice = $this->_invoiceService->prepareInvoice($order);
$invoice->register();
$invoice->save();
$transactionSave = $this->_transaction->addObject(
$invoice
)->addObject(
$invoice->getOrder()
);
$transactionSave->save();
$this->invoiceSender->send($invoice);
//send notification code
$order->addStatusHistoryComment(
__('Notified customer about invoice #%1.', $invoice->getId())
)
->setIsCustomerNotified(true)
->save();
}
}
}
I hope this article is helpful for you. Please leave comment if you have any queries. Thanks!